Generic file I/O module. More...
Data Types | |
interface | CloseFile_deferred |
type | filehandle_base |
class basic (abstract) class for file handles More... | |
interface | filehandle_fortran |
class for Fortran file handle More... | |
type | filehandle_mpi |
class for MPI file handle More... | |
type | fileio_base |
FileIO base class. More... | |
interface | Finalize |
interface | GetBasename_deferred |
interface | GetFilename_deferred |
interface | GetStatus_deferred |
interface | InitFilehandle_deferred |
interface | InitFileIO_deferred |
interface | OpenFile_deferred |
interface | WriteDataset_deferred |
interface | WriteHeader |
Functions/Subroutines | |
type(filehandle_fortran) function | createfilehandle (filename, path, extension, textfile) |
constructor for Fortran file handle More... | |
subroutine | initfilehandle (this, filename, path, extension, textfile, onefile, cycles, unit) |
basic initialization of Fortran file handle More... | |
subroutine | initfileio_base (this, Mesh, Physics, Timedisc, Sources, config, IO, fmtname, fext, textfile) |
Basic FileIO initialization. More... | |
subroutine | writedataset (this, Mesh, Physics, Fluxes, Timedisc, Header, IO) |
Write all data registered for output to the data file. More... | |
subroutine | getendianness (this, res, littlestr, bigstr) |
Determines the endianness of the system. More... | |
pure subroutine | inctime (this) |
Increments the counter for timesteps and sets the time for next output. More... | |
pure subroutine | adjusttimestep (this, time, dt, dtcause) |
Adjust the current timestep. More... | |
subroutine | finalize_base (this) |
Generic destructor of the file I/O. More... | |
subroutine | openfile (this, action, step) |
Open file to access Fortran stream. More... | |
integer function | getunitnumber (this) |
get Fortran i/o unit number More... | |
integer function | getstatus (this, step) |
get Fortran file status More... | |
character(len=16) function | getformat (this) |
get Fortran i/o format string More... | |
pure character(len=fcyclen) function | getstepstring (this, step) |
Get the time step as string with leading zeros. More... | |
character(len=256) function | getbasename (this, step) |
get the file name without path and extension but with step string appended; if thisonefile suppress the time step string More... | |
character(len=256) function | getfilename (this, step) |
Return file name of Fortran stream with full path and extension. More... | |
subroutine | closefile (this, step) |
close Fortran stream More... | |
subroutine | finalize_fortran (this) |
destructor of Fortran stream handle More... | |
subroutine | openfile_mpi (this, action, step) |
Open file for input/ouput in parallel mode. More... | |
subroutine | initfilehandle_mpi (this, filename, path, extension, textfile, onefile, cycles, unit) |
basic initialization of MPI file handle More... | |
integer function | getstatus_mpi (this, step) |
get MPI file status More... | |
subroutine | closefile_mpi (this, step) |
close MPI file More... | |
subroutine | finalize_mpi (this) |
destructor of MPI file handle handle More... | |
Variables | |
integer, save | lastunit = 10 |
Private Attributes | |
file name and extension lengths | |
integer, parameter | fextlen = 4 |
file name extension length More... | |
integer, parameter | fcyclen = 5 |
length of timestep string More... | |
integer, parameter | fnamlen = 256 |
file name length (without any extension) More... | |
integer, parameter | fpatlen = 1024 |
file path length (without file name) More... | |
handling multiple data files with time step in their names | |
integer, parameter | maxcycles = 10000 |
max. number of data files (not counting multiple files per time step in parallel mode) More... | |
Public Attributes | |
file status and access modes | |
integer, parameter | closed = 0 |
file closed More... | |
integer, parameter | readonly = 1 |
readonly access More... | |
integer, parameter | readend = 2 |
readonly access at end More... | |
integer, parameter | replace = 3 |
read/write access replacing file More... | |
integer, parameter | append = 4 |
read/write access at end More... | |
integer, parameter | open_undef = 5 |
open with undefined io action/position More... | |
integer, parameter | fortranfile = 1 |
integer, parameter | mpifile = 2 |
integer, parameter, public | binary = 1 |
file I/O types More... | |
integer, parameter, public | gnuplot = 2 |
integer, parameter, public | vtk = 4 |
integer, parameter, public | xdmf = 7 |
Detailed Description
Generic file I/O module.
This module provides the generic interface routines to all file I/O modules.
Function/Subroutine Documentation
◆ adjusttimestep()
pure subroutine fileio_base_mod::adjusttimestep | ( | class(fileio_base), intent(in) | this, |
real, intent(inout) | time, | ||
real, intent(inout) | dt, | ||
integer, intent(inout) | dtcause | ||
) |
Adjust the current timestep.
Last timestep before output must fit to desired time for output.
- Parameters
-
[in] this [in] this fileio type time [in,out] time dt [in,out] dt timestep dtcause [in,out] dtcause cause of smallest dt
Definition at line 604 of file fileio_base.f90.
◆ closefile()
subroutine fileio_base_mod::closefile | ( | class(filehandle_fortran), intent(inout) | this, |
integer, intent(in) | step | ||
) |
close Fortran stream
- Parameters
-
[in,out] this [in,out] this fileio type [in] step [in] step time step
Definition at line 816 of file fileio_base.f90.

◆ closefile_mpi()
subroutine fileio_base_mod::closefile_mpi | ( | class(filehandle_mpi), intent(inout) | this, |
integer, intent(in) | step | ||
) |
close MPI file
- Parameters
-
[in,out] this [in,out] this fileio type [in] step [in] step time step
Definition at line 991 of file fileio_base.f90.
◆ createfilehandle()
|
private |
constructor for Fortran file handle
- Parameters
-
[in] filename [in] filename file name without extension [in] path [in] path file path without filename [in] extension [in] extension file name extension [in] textfile [in] textfile true for text data
Definition at line 317 of file fileio_base.f90.
◆ finalize_base()
subroutine fileio_base_mod::finalize_base | ( | class(fileio_base), intent(inout) | this | ) |
Generic destructor of the file I/O.
- Parameters
-
[in,out] this [in,out] this fileio type
Definition at line 626 of file fileio_base.f90.
◆ finalize_fortran()
subroutine fileio_base_mod::finalize_fortran | ( | type(filehandle_fortran), intent(inout) | this | ) |
destructor of Fortran stream handle
- Parameters
-
[in,out] this [in,out] this fileio type
Definition at line 845 of file fileio_base.f90.

◆ finalize_mpi()
subroutine fileio_base_mod::finalize_mpi | ( | type(filehandle_mpi), intent(inout) | this | ) |
destructor of MPI file handle handle
- Parameters
-
[in,out] this [in,out] this fileio type
Definition at line 1007 of file fileio_base.f90.

◆ getbasename()
character(len=256) function fileio_base_mod::getbasename | ( | class(filehandle_fortran), intent(in) | this, |
integer, intent(in) | step | ||
) |
get the file name without path and extension but with step string appended; if thisonefile suppress the time step string
- Returns
- file name without path and extension
- Parameters
-
[in] this [in] this file handle [in] step [in] step time step
Definition at line 786 of file fileio_base.f90.

◆ getendianness()
subroutine fileio_base_mod::getendianness | ( | class(fileio_base), intent(inout) | this, |
character(len=*), intent(out) | res, | ||
character(len=*), intent(in) | littlestr, | ||
character(len=*), intent(in) | bigstr | ||
) |
Determines the endianness of the system.
Determines the the endianess of the system (big or little endian)
- Parameters
-
[in,out] this [in,out] this fileio type res [out] res result string littlestr [in] littlestr little endian str bigstr [in] bigstr big endian str
Definition at line 556 of file fileio_base.f90.
◆ getfilename()
character(len=256) function fileio_base_mod::getfilename | ( | class(filehandle_fortran), intent(in) | this, |
integer, intent(in) | step | ||
) |
Return file name of Fortran stream with full path and extension.
- Parameters
-
[in] this [in,out] this fileio type [in] step [in] step time step
Definition at line 803 of file fileio_base.f90.
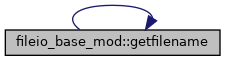

◆ getformat()
character(len=16) function fileio_base_mod::getformat | ( | class(filehandle_fortran), intent(inout) | this | ) |
get Fortran i/o format string
- Parameters
-
[in,out] this [in,out] this fileio type
Definition at line 746 of file fileio_base.f90.
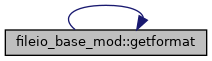
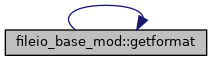
◆ getstatus()
integer function fileio_base_mod::getstatus | ( | class(filehandle_fortran), intent(inout) | this, |
integer, intent(in) | step | ||
) |
get Fortran file status
- Parameters
-
[in,out] this [in,out] this fileio type [in] step [in] step time step
Definition at line 690 of file fileio_base.f90.
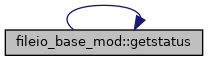

◆ getstatus_mpi()
integer function fileio_base_mod::getstatus_mpi | ( | class(filehandle_mpi), intent(inout) | this, |
integer, intent(in) | step | ||
) |
get MPI file status
- Parameters
-
[in,out] this [in,out] this fileio type [in] step [in] step time step
Definition at line 935 of file fileio_base.f90.
◆ getstepstring()
pure character(len=fcyclen) function fileio_base_mod::getstepstring | ( | class(filehandle_fortran), intent(in) | this, |
integer, intent(in) | step | ||
) |
Get the time step as string with leading zeros.
- Parameters
-
[in] this [in] this file handle [in] step [in] step time step
Definition at line 761 of file fileio_base.f90.
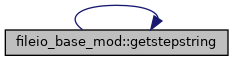
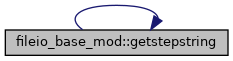
◆ getunitnumber()
integer function fileio_base_mod::getunitnumber | ( | class(filehandle_fortran), intent(inout) | this | ) |
get Fortran i/o unit number
- Parameters
-
[in,out] this [in,out] this fileio type
Definition at line 679 of file fileio_base.f90.
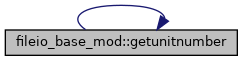
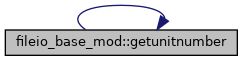
◆ inctime()
pure subroutine fileio_base_mod::inctime | ( | class(fileio_base), intent(inout) | this | ) |
Increments the counter for timesteps and sets the time for next output.
- Parameters
-
[in,out] this [in,out] this fileio type
Definition at line 591 of file fileio_base.f90.
◆ initfilehandle()
|
private |
basic initialization of Fortran file handle
- Parameters
-
[in,out] this [inout] this file handle class [in] filename [in] filename file name without extension [in] path [in] path file path without filename [in] extension [in] extension file name extension [in] textfile [in] textfile true for text data [in] onefile [in] onefile true if all data goes into one file [in] cycles [in] cycles max number of files [in] unit [in] unit force fortran i/o unit number
Definition at line 330 of file fileio_base.f90.

◆ initfilehandle_mpi()
|
private |
basic initialization of MPI file handle
- Parameters
-
[in,out] this [inout] this file handle class [in] filename [in] filename file name without extension [in] path [in] path file path without filename [in] extension [in] extension file name extension [in] textfile [in] textfile true for text data [in] onefile [in] onefile true if all data goes into one file [in] cycles [in] cycles max number of files [in] unit [in] unit force fortran i/o unit number
Definition at line 916 of file fileio_base.f90.
◆ initfileio_base()
subroutine fileio_base_mod::initfileio_base | ( | class(fileio_base), intent(inout) | this, |
class(mesh_base), intent(in) | Mesh, | ||
class(physics_base), intent(in) | Physics, | ||
class(timedisc_base), intent(in) | Timedisc, | ||
class(sources_list), intent(in), allocatable | Sources, | ||
type(dict_typ), intent(in), pointer | config, | ||
type(dict_typ), intent(in), pointer | IO, | ||
character(len=*), intent(in) | fmtname, | ||
character(len=*), intent(in) | fext, | ||
logical, intent(in), optional | textfile | ||
) |
Basic FileIO initialization.
- Parameters
-
[in,out] this [in,out] this fileio type [in] mesh [in] Mesh mesh type [in] physics [in] Physics physics type [in] timedisc [in] Timedisc timedisc type [in] sources [in] Sources sources type [in] config [in] config dict with I/O configuration [in] io [in] IO dict with pointers to I/O arrays [in] fmtname [in] fmtname file format [in] fext [in] fext file extension [in] textfile [in] textfile true for text data
Definition at line 390 of file fileio_base.f90.

◆ openfile()
subroutine fileio_base_mod::openfile | ( | class(filehandle_fortran), intent(inout) | this, |
integer, intent(in) | action, | ||
integer, intent(in) | step | ||
) |
Open file to access Fortran stream.
- Parameters
-
[in,out] this [in,out] this fileio type [in] action [in] action mode of file access [in] step [in] step time step
Definition at line 638 of file fileio_base.f90.

◆ openfile_mpi()
subroutine fileio_base_mod::openfile_mpi | ( | class(filehandle_mpi), intent(inout) | this, |
integer, intent(in) | action, | ||
integer, intent(in) | step | ||
) |
Open file for input/ouput in parallel mode.
- Parameters
-
[in,out] this [in,out] this fileio type [in] action [in] action mode of file access [in] step [in] step time step
Definition at line 859 of file fileio_base.f90.
◆ writedataset()
subroutine fileio_base_mod::writedataset | ( | class(fileio_base), intent(inout) | this, |
class(mesh_base), intent(in) | Mesh, | ||
class(physics_base), intent(inout) | Physics, | ||
class(fluxes_base), intent(in) | Fluxes, | ||
class(timedisc_base), intent(in) | Timedisc, | ||
type(dict_typ), pointer | Header, | ||
type(dict_typ), pointer | IO | ||
) |
Write all data registered for output to the data file.
- Parameters
-
[in,out] this [in,out] this fileio type [in] mesh [in] mesh mesh type [in,out] physics [in] physics physics type [in] fluxes [in] fluxes fluxes type [in] timedisc [in] timedisc timedisc type io [in,out] IO I/O dictionary
Definition at line 514 of file fileio_base.f90.
Variable Documentation
◆ append
|
private |
read/write access at end
Definition at line 277 of file fileio_base.f90.
◆ binary
integer, parameter, public fileio_base_mod::binary = 1 |
file I/O types
Definition at line 286 of file fileio_base.f90.
◆ closed
|
private |
file closed
Definition at line 273 of file fileio_base.f90.
◆ fcyclen
|
private |
length of timestep string
Definition at line 80 of file fileio_base.f90.
◆ fextlen
|
private |
file name extension length
Definition at line 79 of file fileio_base.f90.
◆ fnamlen
|
private |
file name length (without any extension)
Definition at line 81 of file fileio_base.f90.
◆ fortranfile
|
private |
file formats
Definition at line 283 of file fileio_base.f90.
◆ fpatlen
|
private |
file path length (without file name)
Definition at line 82 of file fileio_base.f90.
◆ gnuplot
integer, parameter, public fileio_base_mod::gnuplot = 2 |
Definition at line 287 of file fileio_base.f90.
◆ lastunit
|
private |
Definition at line 294 of file fileio_base.f90.
◆ maxcycles
|
private |
max. number of data files (not counting multiple files per time step in parallel mode)
Definition at line 85 of file fileio_base.f90.
◆ mpifile
|
private |
Definition at line 284 of file fileio_base.f90.
◆ open_undef
|
private |
open with undefined io action/position
Definition at line 278 of file fileio_base.f90.
◆ readend
|
private |
readonly access at end
Definition at line 275 of file fileio_base.f90.
◆ readonly
|
private |
readonly access
Definition at line 274 of file fileio_base.f90.
◆ replace
|
private |
read/write access replacing file
Definition at line 276 of file fileio_base.f90.
◆ vtk
integer, parameter, public fileio_base_mod::vtk = 4 |
Definition at line 288 of file fileio_base.f90.
◆ xdmf
integer, parameter, public fileio_base_mod::xdmf = 7 |
Definition at line 291 of file fileio_base.f90.