Classes | |
class | DomainMap |
class | Grid |
class | InvalidIndexError |
class | StreamMask |
class | StreamplotSet |
class | TerminateTrajectory |
Functions | |
def | streamplot (axes, x, y, u, v, density=1, linewidth=None, color=None, cmap=None, norm=None, arrowsize=1, arrowstyle='-|>', minlength=0.1, maxlength=4.0, transform=None, zorder=2, start_points=None, integration_direction='both') |
def | get_integrator (u, v, dmap, minlength, maxlength, integration_direction) |
def | _integrate_rk12 (x0, y0, dmap, f, maxlength) |
def | _euler_step (xf_traj, yf_traj, dmap, f) |
def | interpgrid (a, xi, yi) |
def | _gen_starting_points (shape) |
Variables | |
list | __all__ = ['streamplot'] |
Detailed Description
Streamline plotting for 2D vector fields.
Function Documentation
◆ _euler_step()
|
private |
Simple Euler integration step that extends streamline to boundary.
Definition at line 556 of file streamplot.py.
Here is the call graph for this function:

Here is the caller graph for this function:

◆ _gen_starting_points()
|
private |
Yield starting points for streamlines. Trying points on the boundary first gives higher quality streamlines. This algorithm starts with a point on the mask corner and spirals inward. This algorithm is inefficient, but fast compared to rest of streamplot.
Definition at line 623 of file streamplot.py.
Here is the caller graph for this function:

◆ _integrate_rk12()
|
private |
2nd-order Runge-Kutta algorithm with adaptive step size. This method is also referred to as the improved Euler's method, or Heun's method. This method is favored over higher-order methods because: 1. To get decent looking trajectories and to sample every mask cell on the trajectory we need a small timestep, so a lower order solver doesn't hurt us unless the data is *very* high resolution. In fact, for cases where the user inputs data smaller or of similar grid size to the mask grid, the higher order corrections are negligible because of the very fast linear interpolation used in `interpgrid`. 2. For high resolution input data (i.e. beyond the mask resolution), we must reduce the timestep. Therefore, an adaptive timestep is more suited to the problem as this would be very hard to judge automatically otherwise. This integrator is about 1.5 - 2x as fast as both the RK4 and RK45 solvers in most setups on my machine. I would recommend removing the other two to keep things simple.
Definition at line 464 of file streamplot.py.
Here is the call graph for this function:

◆ get_integrator()
def streamplot.get_integrator | ( | u, | |
v, | |||
dmap, | |||
minlength, | |||
maxlength, | |||
integration_direction | |||
) |
◆ interpgrid()
def streamplot.interpgrid | ( | a, | |
xi, | |||
yi | |||
) |
Fast 2D, linear interpolation on an integer grid
Definition at line 583 of file streamplot.py.
Here is the caller graph for this function:

◆ streamplot()
def streamplot.streamplot | ( | axes, | |
x, | |||
y, | |||
u, | |||
v, | |||
density = 1 , |
|||
linewidth = None , |
|||
color = None , |
|||
cmap = None , |
|||
norm = None , |
|||
arrowsize = 1 , |
|||
arrowstyle = '-|>' , |
|||
minlength = 0.1 , |
|||
maxlength = 4.0 , |
|||
transform = None , |
|||
zorder = 2 , |
|||
start_points = None , |
|||
integration_direction = 'both' |
|||
) |
Draws streamlines of a vector flow. *x*, *y* : 1d arrays an *evenly spaced* grid. *u*, *v* : 2d arrays x and y-velocities. Number of rows should match length of y, and the number of columns should match x. *density* : float or 2-tuple Controls the closeness of streamlines. When `density = 1`, the domain is divided into a 30x30 grid---*density* linearly scales this grid. Each cell in the grid can have, at most, one traversing streamline. For different densities in each direction, use [density_x, density_y]. *linewidth* : numeric or 2d array vary linewidth when given a 2d array with the same shape as velocities. *color* : matplotlib color code, or 2d array Streamline color. When given an array with the same shape as velocities, *color* values are converted to colors using *cmap*. *cmap* : :class:`~matplotlib.colors.Colormap` Colormap used to plot streamlines and arrows. Only necessary when using an array input for *color*. *norm* : :class:`~matplotlib.colors.Normalize` Normalize object used to scale luminance data to 0, 1. If None, stretch (min, max) to (0, 1). Only necessary when *color* is an array. *arrowsize* : float Factor scale arrow size. *arrowstyle* : str Arrow style specification. See :class:`~matplotlib.patches.FancyArrowPatch`. *minlength* : float Minimum length of streamline in axes coordinates. *maxlength* : float Maximum length of streamline in axes coordinates. *start_points*: Nx2 array Coordinates of starting points for the streamlines. In data coordinates, the same as the ``x`` and ``y`` arrays. *integration_direction* : ['foward','backward','both'] Integrate the streamline in forward, backward or both directions. *zorder* : int any number Returns: *stream_container* : StreamplotSet Container object with attributes - lines: `matplotlib.collections.LineCollection` of streamlines - arrows: collection of `matplotlib.patches.FancyArrowPatch` objects representing arrows half-way along stream lines. This container will probably change in the future to allow changes to the colormap, alpha, etc. for both lines and arrows, but these changes should be backward compatible.
Definition at line 22 of file streamplot.py.
Here is the call graph for this function:
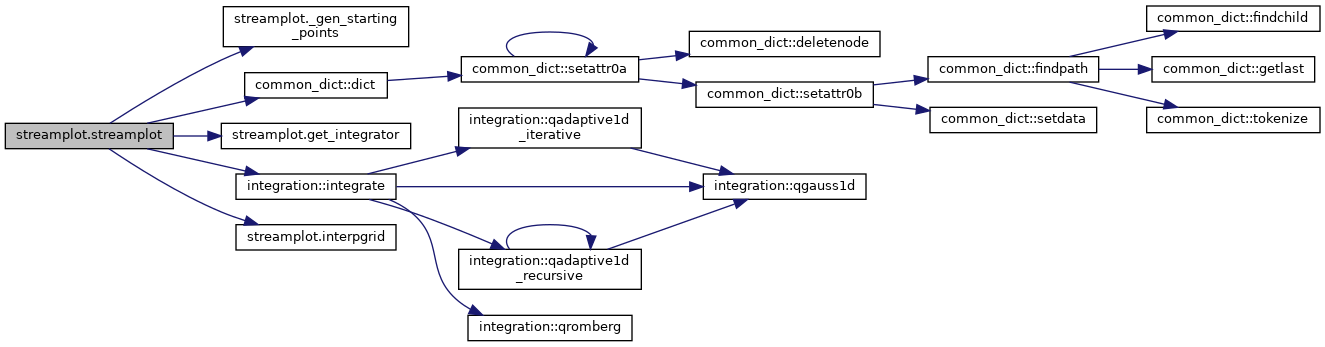
Variable Documentation
◆ __all__
|
private |
Definition at line 19 of file streamplot.py.