module for binary file I/O More...
Data Types | |
type | fileio_binary |
FileIO binary class. More... | |
Variables | |
character, parameter | lf = ACHAR(10) |
line feed More... | |
Methods | |
subroutine | initfileio_binary (this, Mesh, Physics, Timedisc, Sources, config, IO) |
Constructor for the binary file I/O. More... | |
subroutine | writeheader (this, Mesh, Physics, Header, IO) |
Write the file header The header is written in ASCII and is 13 Byte long. First a "magic" identifier is written, than the endianness (II=little, MM=big), a single byte file format version number, two char realsize, two char intsize. This would result for example to (\0=hex 0): "FOSITEII\0 8 4". More... | |
subroutine | writekey (this, key, type, bytes, dims) |
Writes key structure This subroutine writes the the key, data type and data sizes. It is defined as following (suppose 4B Integer) | 4B length of key | *B key | 4B data type | 4B data size in bytes | If the data type is a 2D,3D or 4D array, 2, 3 or 4 (4 Byte) integers are appended with the shape information. There storage is included in the data size field. Therefore without knowing the data types, one can jump over the data to the next key structure. More... | |
recursive subroutine | writedataattributes (this, Mesh, config, path) |
Writes data attributes to a file. More... | |
subroutine | writenode (this, Mesh, key, node) |
logical function | hasmeshdims (this, Mesh, dims) |
logical function | hascornerdims (this, Mesh, dims) |
subroutine | setoutputdims (this, Mesh, dims) |
subroutine | writedataset_binary (this, Mesh, Physics, Fluxes, Timedisc, Header, IO) |
Writes all desired data arrays to a file. More... | |
subroutine | finalize (this) |
Closes the file I/O. More... | |
Detailed Description
module for binary file I/O
This module implements file I/O, which writes all of the output array in a self describing binary data format including informations about endianness and precision.
Specification: [header],[data],[data],[data],..
- header: [magic],[endian],[version],[real size],[integer size] 6 + 2 + 1 + 2 + 2 = 13 bytes These are all ASCII characters except the version, which is single byte unsigned integer.
- data: [key length],[key],[type],[data length],[[dims]],[[data]] 4 * 4 4 * * bytes If [type] indicates a 2D, 3D, 4D or 5D array, [data length] includes 8, 12, 16 or 24 bytes extra, for dimensional information. The ASCII [key] has the in [key length] specified size. The different [type]s are defined in common/common_dict.f90.
To write the binary files, we need one of the following:
- a fortran compiler with f2003 Stream IO
- a MPI build
- on NEC sx9: Set the runtime enviroment variable F_NORCW=5555 (or to another value)
Function/Subroutine Documentation
◆ finalize()
subroutine fileio_binary_mod::finalize | ( | type(fileio_binary), intent(inout) | this | ) |
Closes the file I/O.
Definition at line 672 of file fileio_binary.f90.
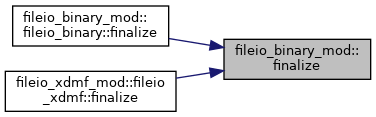
◆ hascornerdims()
|
private |
- Parameters
-
[in,out] this [in] this type [in] mesh [in] mesh mesh type
Definition at line 595 of file fileio_binary.f90.
◆ hasmeshdims()
|
private |
- Parameters
-
[in,out] this [in] this type [in] mesh [in] mesh mesh type [in] dims [in] dims array dimensions
Definition at line 577 of file fileio_binary.f90.
◆ initfileio_binary()
subroutine fileio_binary_mod::initfileio_binary | ( | class(fileio_binary), intent(inout) | this, |
class(mesh_base), intent(in) | Mesh, | ||
class(physics_base), intent(in) | Physics, | ||
class(timedisc_base), intent(in) | Timedisc, | ||
class(sources_list), intent(in), allocatable | Sources, | ||
type(dict_typ), intent(in), pointer | config, | ||
type(dict_typ), intent(in), pointer | IO | ||
) |
Constructor for the binary file I/O.
Initilizes the file I/O type, filename, stoptime, number of outputs, number of files, unit number, config as a dict
- Parameters
-
[in,out] this [in,out] this fileio type [in] mesh [in] Mesh mesh type [in] physics [in] Physics Physics type [in] timedisc [in] Timedisc timedisc type [in] sources [in] Sources sources type [in] io [in] IO Dictionary for I/O
Definition at line 129 of file fileio_binary.f90.
◆ setoutputdims()
|
private |
Definition at line 616 of file fileio_binary.f90.
◆ writedataattributes()
|
private |
Writes data attributes to a file.
- Parameters
-
[in,out] this [in,out] this fileio type [in] mesh [in] mesh mesh type config [in] config dict of configuration path [in] path
Definition at line 367 of file fileio_binary.f90.
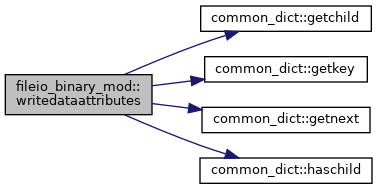
◆ writedataset_binary()
subroutine fileio_binary_mod::writedataset_binary | ( | class(fileio_binary), intent(inout) | this, |
class(mesh_base), intent(in) | Mesh, | ||
class(physics_base), intent(inout) | Physics, | ||
class(fluxes_base), intent(in) | Fluxes, | ||
class(timedisc_base), intent(in) | Timedisc, | ||
type(dict_typ), pointer | Header, | ||
type(dict_typ), pointer | IO | ||
) |
Writes all desired data arrays to a file.
- Parameters
-
[in,out] this [in,out] this fileio type [in] mesh [in] mesh mesh type [in,out] physics [in] physics physics type [in] fluxes [in] fluxes fluxes type [in] timedisc [in] timedisc timedisc type io [in,out] IO I/O dictionary
Definition at line 638 of file fileio_binary.f90.
◆ writeheader()
subroutine fileio_binary_mod::writeheader | ( | class(fileio_binary), intent(inout) | this, |
class(mesh_base), intent(in) | Mesh, | ||
class(physics_base), intent(in) | Physics, | ||
type(dict_typ), pointer | Header, | ||
type(dict_typ), pointer | IO | ||
) |
Write the file header The header is written in ASCII and is 13 Byte long. First a "magic" identifier is written, than the endianness (II=little, MM=big), a single byte file format version number, two char realsize, two char intsize. This would result for example to (\0=hex 0): "FOSITEII\0 8 4".
- Parameters
-
[in,out] this [in,out] this fileio type [in] mesh [in] Mesh mesh type [in] physics [in] Physics physics type
Definition at line 215 of file fileio_binary.f90.
◆ writekey()
|
private |
Writes key structure This subroutine writes the the key, data type and data sizes. It is defined as following (suppose 4B Integer) | 4B length of key | *B key | 4B data type | 4B data size in bytes | If the data type is a 2D,3D or 4D array, 2, 3 or 4 (4 Byte) integers are appended with the shape information. There storage is included in the data size field. Therefore without knowing the data types, one can jump over the data to the next key structure.
- Parameters
-
[in,out] this [in,out] this fileio type
Definition at line 298 of file fileio_binary.f90.
◆ writenode()
|
private |
- Parameters
-
[in,out] this [in,out] this fileio type [in] mesh [in] mesh mesh type node [in] data node
Definition at line 398 of file fileio_binary.f90.
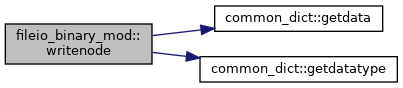
Variable Documentation
◆ lf
|
private |
line feed
Definition at line 83 of file fileio_binary.f90.