basic mesh module More...
Data Types | |
type | fargo_base |
base class for fargo transport properties and methods More... | |
interface | Finalize |
type | mesh_base |
mesh data structure More... | |
interface | TensorDivergence2D_1 |
interface | TensorDivergence3D |
interface | VectorDivergence2D_1 |
interface | VectorDivergence3D |
Public Attributes | |
mesh types | |
integer, parameter | midpoint = 1 |
use midpoint rule to approximate flux integrals More... | |
integer, dimension(3), parameter | nfaces = (/ 2, 4, 6 /) |
number of faces More... | |
integer, dimension(3), parameter | ncorners = (/ 2, 4, 8 /) |
number of corners More... | |
integer, parameter | west = 1 |
named constant for western boundary More... | |
integer, parameter | east = 2 |
named constant for eastern boundary More... | |
integer, parameter | south = 3 |
named constant for southern boundary More... | |
integer, parameter | north = 4 |
named constant for northern boundary More... | |
integer, parameter | bottom = 5 |
named constant for bottom boundary More... | |
integer, parameter | top = 6 |
named constant for top boundary More... | |
integer, parameter | vector_x = INT(B'001') |
flags to check which vector components are enabled More... | |
integer, parameter | vector_y = INT(B'010') |
integer, parameter | vector_z = INT(B'100') |
Variables in Parallel Mode | |
subroutine | initmesh (this, config, IO, mtype, mname) |
Constructor of generic mesh module. More... | |
subroutine | setoutput (this, config, IO) |
Setup mesh fields for i/o. More... | |
subroutine | calculaterotation (this) |
initialize array for rotation angle More... | |
pure logical function | internalpoint (this, x, y, z, mask) |
Check if a given coordinate pair represents an internal point. More... | |
subroutine | initmesh_parallel (this, config) |
Initialize MPI (parallel mode only) More... | |
subroutine | calculatedecomposition (ni, nj, nk, ginum, pi, pj, pk) |
return the best partitioning of processes More... | |
real function, dimension(:,:,:), pointer | remapbounds_1 (this, array) |
remap lower bounds in the first 2 dimensions of rank 2 subarrays More... | |
real function, dimension(:,:,:,:), pointer | remapbounds_2 (this, array) |
remap lower bounds in the first 2 dimensions of rank 3 subarrays More... | |
real function, dimension(:,:,:,:,:), pointer | remapbounds_3 (this, array) |
remap lower bounds in the first 2 dimensions of rank 4 subarrays More... | |
real function, dimension(:,:,:,:,:,:), pointer | remapbounds_4 (this, array) |
remap lower bounds in the first 2 dimensions of rank 5 subarrays More... | |
subroutine | finalize_base (this) |
Destructor of mesh class. More... | |
pure integer function | getdirection (this) |
Get the fargo transport direction. More... | |
pure character(len=4) function | getdirectionname (this) |
Get the fargo transport direction as string. More... | |
Detailed Description
basic mesh module
Function/Subroutine Documentation
◆ calculatedecomposition()
|
private |
return the best partitioning of processes
compute pi x pj x pk for a given mesh with resolution ni x nj x nk and number of ghost cells ginum in the 1st dimension (used for optimizing vector length) pi = pnum initially with total number of processes pnum<MAXNUM (see module "factors") pj, pk = 1 initially pk returns 0, for erroneous input
Definition at line 1320 of file mesh_base.f90.


◆ calculaterotation()
|
private |
initialize array for rotation angle
the rotation angle contains the local angle between the direction of the curve along which the second curvilinear coordinate varies (coordinate curve along the 1st curvilinear coordinate) and the cartesian x-direction (coordinate curve along the 1st cartesian coordinate)
- Parameters
-
this [in,out] this all mesh data
Definition at line 935 of file mesh_base.f90.
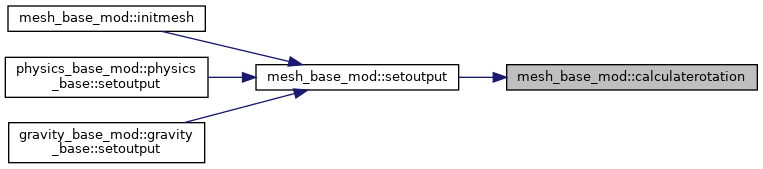
◆ finalize_base()
subroutine mesh_base_mod::finalize_base | ( | class(mesh_base), intent(inout) | this | ) |
Destructor of mesh class.
- Parameters
-
[in,out] this [in,out] this all mesh data
Definition at line 1548 of file mesh_base.f90.

◆ getdirection()
pure integer function mesh_base_mod::getdirection | ( | class(fargo_base), intent(in) | this | ) |
Get the fargo transport direction.
- Returns
- coordinate direction of fargo transport, i.e. {1,2,3} or 0 if disabled
- Parameters
-
[in] this [in] this fargo type
Definition at line 1577 of file mesh_base.f90.
◆ getdirectionname()
pure character(len=4) function mesh_base_mod::getdirectionname | ( | class(fargo_base), intent(in) | this | ) |
Get the fargo transport direction as string.
- Returns
- coordinate direction of fargo transport, i.e. {x,y,z} or none if disabled
- Parameters
-
[in] this [in] this fargo type
Definition at line 1588 of file mesh_base.f90.
◆ initmesh()
subroutine mesh_base_mod::initmesh | ( | class(mesh_base), intent(inout) | this, |
type(dict_typ), pointer | config, | ||
type(dict_typ), pointer | IO, | ||
integer | mtype, | ||
character(len=32) | mname | ||
) |
Constructor of generic mesh module.
This subroutine reads the necessary config data for setting up the mesh. It initializes the geometry and various mesh data arrays. Some of those are marked for output. For a detailed discription of the various geometries and how to setup those see geometry .
- Parameters
-
this [in,out] this all mesh data config [in,out] config sub-dictionary with mesh configuration data io [in,out] IO mesh specific i/o data
- Todo:
- check if min/max coordinates are compatible with the restrictions imposed by the geometry
Definition at line 295 of file mesh_base.f90.

◆ initmesh_parallel()
subroutine mesh_base_mod::initmesh_parallel | ( | class(mesh_base), intent(inout) | this, |
type(dict_typ), pointer | config | ||
) |
Initialize MPI (parallel mode only)
Create a 3D cartesian topology of processes and subdivide the computational domain. ATTENTION: The dimensionality of the process topology is always 3D even for 1D/2D simulations!
- Parameters
-
[in,out] this [in,out] this all mesh data
Definition at line 1022 of file mesh_base.f90.


◆ internalpoint()
pure logical function mesh_base_mod::internalpoint | ( | class(mesh_base), intent(in) | this, |
real, intent(in) | x, | ||
real, intent(in) | y, | ||
real, intent(in) | z, | ||
integer, dimension(6), intent(in), optional | mask | ||
) |
Check if a given coordinate pair represents an internal point.
This function checks if a point given by its curvilinear coordinates (x,y) lies inside the computational domain or (if mask is given) inside a rectangular (with respect to the curvilinear mesh) region specified by an index mask.
- Parameters
-
[in] this [in,out] this all mesh data
Definition at line 961 of file mesh_base.f90.
◆ remapbounds_1()
real function, dimension(:,:,:), pointer mesh_base_mod::remapbounds_1 | ( | class(mesh_base) | this, |
real, dimension(this%igmin:,this%jgmin:,this%kgmin:), intent(in), target | array | ||
) |
remap lower bounds in the first 2 dimensions of rank 2 subarrays
This is a short hack to obviate a restriction in the generation of subarray pointers. In Fortran 90/95 the indices of subarrays always start with a lower bound of 1, but Fosite requires that all mesh data arrays start with lower bounds of IGMIN and JGMIN, which are not equal to 1 in general.
- Parameters
-
this [in,out] this all mesh data
Definition at line 1488 of file mesh_base.f90.
◆ remapbounds_2()
real function, dimension(:,:,:,:), pointer mesh_base_mod::remapbounds_2 | ( | class(mesh_base) | this, |
real, dimension(this%igmin:,this%jgmin:,this%kgmin:,:), intent(in), target | array | ||
) |
remap lower bounds in the first 2 dimensions of rank 3 subarrays
- Parameters
-
this [in,out] this all mesh data
Definition at line 1502 of file mesh_base.f90.
◆ remapbounds_3()
real function, dimension(:,:,:,:,:), pointer mesh_base_mod::remapbounds_3 | ( | class(mesh_base) | this, |
real, dimension(this%igmin:,this%jgmin:,this%kgmin:,:,:), intent(in), target | array | ||
) |
remap lower bounds in the first 2 dimensions of rank 4 subarrays
- Parameters
-
this [in,out] this all mesh data
Definition at line 1517 of file mesh_base.f90.

◆ remapbounds_4()
real function, dimension(:,:,:,:,:,:), pointer mesh_base_mod::remapbounds_4 | ( | class(mesh_base) | this, |
real, dimension(this%igmin:,this%jgmin:,this%kgmin:,:,:,:), intent(in), target | array | ||
) |
remap lower bounds in the first 2 dimensions of rank 5 subarrays
- Parameters
-
this [in,out] this all mesh data
Definition at line 1532 of file mesh_base.f90.

◆ setoutput()
|
private |
Setup mesh fields for i/o.
- Parameters
-
[in,out] this [in,out] this all mesh data
Definition at line 827 of file mesh_base.f90.

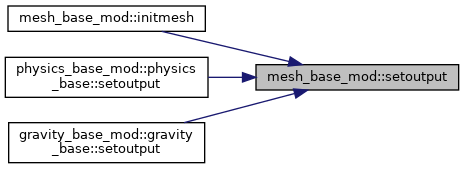
Variable Documentation
◆ bottom
|
private |
named constant for bottom boundary
Definition at line 101 of file mesh_base.f90.
◆ east
|
private |
named constant for eastern boundary
Definition at line 101 of file mesh_base.f90.
◆ midpoint
|
private |
use midpoint rule to approximate flux integrals
Definition at line 95 of file mesh_base.f90.
◆ ncorners
|
private |
number of corners
Definition at line 99 of file mesh_base.f90.
◆ nfaces
|
private |
number of faces
Definition at line 98 of file mesh_base.f90.
◆ north
|
private |
named constant for northern boundary
Definition at line 101 of file mesh_base.f90.
◆ south
|
private |
named constant for southern boundary
Definition at line 101 of file mesh_base.f90.
◆ top
|
private |
named constant for top boundary
Definition at line 101 of file mesh_base.f90.
◆ vector_x
|
private |
flags to check which vector components are enabled
Definition at line 109 of file mesh_base.f90.
◆ vector_y
|
private |
Definition at line 109 of file mesh_base.f90.
◆ vector_z
|
private |
Definition at line 109 of file mesh_base.f90.
◆ west
|
private |
named constant for western boundary
- Todo:
- Check why this is not in boundary base?!
Definition at line 101 of file mesh_base.f90.